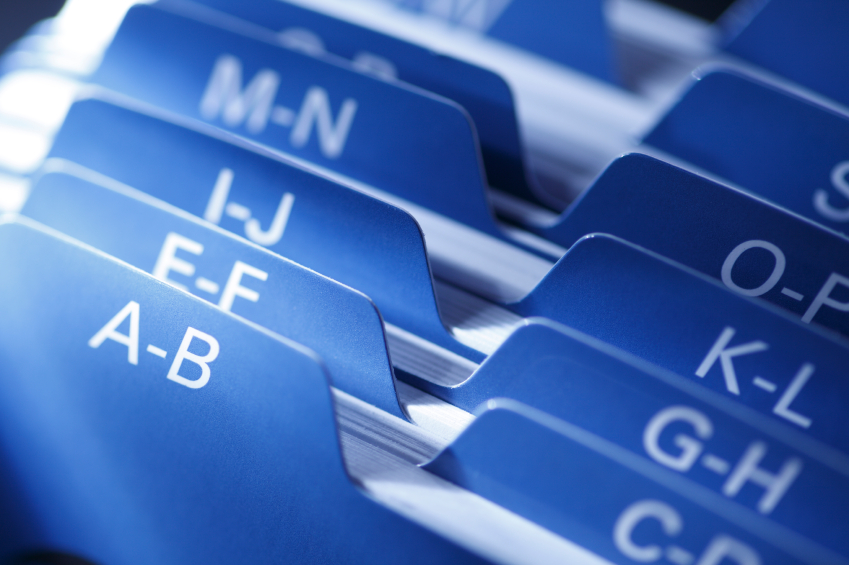
Techrens Alert – part 6
Moving right along let’s make it better to input the contact, because we want to work smarter not harder.
Page 6
The first thing we need to do is add a permission to allow us to read the contacts.
<uses-permission android:name=”android.permission.READ_CONTACTS” />
Next I updated the content_testt1.xml by adding a button label Get Contact with a ID of btnGetContact.
Now I updated the TestActivity with the following code (see blue highlights for changes)
public class TestActivity1 extends AppCompatActivity implements LocationListener { LocationManager locationManager; String mprovider; Location location; TextView message; EditText number; private static final int REQUEST_CODE_PICK_CONTACTS = 1; private Uri uriContact; private String contactID; // contacts unique ID @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_test1); Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar); setSupportActionBar(toolbar); message = (TextView) findViewById(R.id.txtMessage); number = (EditText) findViewById(R.id.txtNumber); FloatingActionButton fab = (FloatingActionButton) findViewById(R.id.fab); fab.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Snackbar.make(view, "Replace with your own action", Snackbar.LENGTH_LONG) .setAction("Action", null).show(); } }); final Button button = (Button) findViewById(R.id.btnCheckGps); button.setOnClickListener(new View.OnClickListener() { public void onClick(View v) { getLocation(); } }); final Button openLink = (Button) findViewById(R.id.btnOpenLink); openLink.setOnClickListener(new View.OnClickListener() { public void onClick(View v) { if (location != null) { getGeoInfo(); getUrl(); } // } }); final Button getContact = (Button) findViewById(R.id.btnGetContact); getContact.setOnClickListener(new View.OnClickListener() { public void onClick(View v) { startActivityForResult(new Intent(Intent.ACTION_PICK, ContactsContract.Contacts.CONTENT_URI), REQUEST_CODE_PICK_CONTACTS); } }); } private void retrieveContactNumber() { String contactNumber = null; // getting contacts ID Cursor cursorID = getContentResolver().query(uriContact, new String[]{ContactsContract.Contacts._ID}, null, null, null); if (cursorID.moveToFirst()) { contactID = cursorID.getString(cursorID.getColumnIndex(ContactsContract.Contacts._ID)); } cursorID.close(); //Log.d(TAG, "Contact ID: " + contactID); // Using the contact ID now we will get contact phone number Cursor cursorPhone = getContentResolver().query(ContactsContract.CommonDataKinds.Phone.CONTENT_URI, new String[]{ContactsContract.CommonDataKinds.Phone.NUMBER}, ContactsContract.CommonDataKinds.Phone.CONTACT_ID + " = ? AND " + ContactsContract.CommonDataKinds.Phone.TYPE + " = " + ContactsContract.CommonDataKinds.Phone.TYPE_MOBILE, new String[]{contactID}, null); if (cursorPhone.moveToFirst()) { contactNumber = cursorPhone.getString(cursorPhone.getColumnIndex(ContactsContract.CommonDataKinds.Phone.NUMBER)); number.setText(contactNumber); } cursorPhone.close(); //Log.d(TAG, "Contact Phone Number: " + contactNumber); } private void getGeoInfo(){ Geocoder geocoder; List<Address> addresses; geocoder = new Geocoder(this, Locale.getDefault()); try { addresses = geocoder.getFromLocation(location.getLatitude(), location.getLongitude(), 1); // Here 1 represent max location result to returned, by documents it recommended 1 to 5 String address = addresses.get(0).getAddressLine(0); // If any additional address line present than only, check with max available address lines by getMaxAddressLineIndex() String city = addresses.get(0).getLocality(); String state = addresses.get(0).getAdminArea(); String country = addresses.get(0).getCountryName(); String postalCode = addresses.get(0).getPostalCode(); String knownName = addresses.get(0).getFeatureName(); // Only if available else return NULL String addressText = address + " " + city + ", " + state; message.setText(addressText); String iNumber = number.getText().toString(); if (iNumber != null && !iNumber.isEmpty() && !iNumber.equals("null")) { SmsManager smsManager = SmsManager.getDefault(); smsManager.sendTextMessage(number.getText().toString(), null, "I'm at " + addressText + " " + "maps.google.com/maps?q=" + location.getLatitude() + "," + location.getLongitude(), null, null); } Toast.makeText(getBaseContext(), addressText, Toast.LENGTH_LONG).show(); } catch (IOException e) { } } private void getUrl(){ String myUrl = "http://maps.google.com/maps?q=" + location.getLatitude() + "," + location.getLongitude(); Intent browserIntent = new Intent(Intent.ACTION_VIEW, Uri.parse(myUrl)); startActivity(browserIntent); } private void getLocation() { locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE); Criteria criteria = new Criteria(); mprovider = locationManager.getBestProvider(criteria, false); if (mprovider != null && !mprovider.equals("")) { if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) { return; } location = locationManager.getLastKnownLocation(mprovider); locationManager.requestLocationUpdates(mprovider, 15000, 1, this); if (location != null) onLocationChanged(location); else Toast.makeText(getBaseContext(), "No Location Provider Found Check Your Code", Toast.LENGTH_SHORT).show(); } } @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { super.onActivityResult(requestCode, resultCode, data); if (requestCode == REQUEST_CODE_PICK_CONTACTS && resultCode == RESULT_OK) { //Log.d(TAG, "Response: " + data.toString()); uriContact = data.getData(); retrieveContactNumber(); } } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.menu_test_activity1, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId(); //noinspection SimplifiableIfStatement if (id == R.id.action_settings) { return true; } return super.onOptionsItemSelected(item); } @Override public void onLocationChanged(Location location) { message.setText("Current Longitude:" + location.getLongitude() + "\n\nCurrent Latitude:" + location.getLatitude()); } @Override public void onStatusChanged(String s, int i, Bundle bundle) { } @Override public void onProviderEnabled(String s) { } @Override public void onProviderDisabled(String s) { } }
First I define three variables that I will need for selecting the contacts.
- REQUEST_CODE_PICK_CONTACTS
- uriContact
- contactID
I then initialize the get button and set an intent for its on click listener. I then create a method called retrieveContactNumber to retrieve contact information. Once the user selects a contact it calls the retrieveContactNumber which selects the mobile phone number. Once I have the mobile phone number I then it is placed on the number text box.
So there you go, a good foundation if I do say so myself! 😉
Here is the apk if you would like to test:
Please leave comments below, I would love to hear thoughts on this process!